CSS Fade In Transition: Text, Images, Scroll & Hover
CSS transitions allow HTML elements within a web page to gradually change from one state to another. A popular scenario is defining a quick set of properties that provides clean animations to the visual elements of your web page.
You can easily adjust an element's transparency with a fade in transition using CSS by utilizing smooth animation techniques and very little code. In this case, we'll be using CSS transitions to change the opacity
rule within a set timeframe.
How CSS Transitions Work
There are a few rules we'll be following:
opacity
: Sets the visual transparency of the element, with accepted values ranging from 0.0 (fully transparent) to 1.0 (fully opaque).transition
: This is the shortened version of many different transition properties where1s
is the duration of the transition effect, in this case one second,all
specifics the property that will be animated (in this case, all properties of the element), andease-in-out
specifies the speed curve for the transition, which in this case means the animation will speed up midway through and slow down before the animation completes.
Note that certain CSS rules, including the transition
rule, come with a set of vendor-specific prefixes we can use for a variety of browsers, including -webkit
for Chrome and Safari browsers and -moz
for Firefox browsers. You could also include -ms
for Microsoft browsers like Internet Explorer, even though Microsoft no longer supports this browser, or -o
for the Opera browser.
The Fade In Transition
Let's start with a basic div
element:
<div class="fade-in"></div>
And some styling to differentiate our new element from the rest of the page:
.fade-in {
width: 100px;
height: 100px;
background-color: red;
cursor: pointer;
}
The above CSS code styles our new element with class fade-in
by making it 100x100 pixels in size with a red background. cursor: pointer
sets the mouse cursor to the pointer graphic so we know when we've hovered over our .fade-in
element on the page.
This also means that any HTML element with class .fade-in
will perform the animation we're about to set up.
Now let's update that styling a bit to include some transition rules and a fade in effect on mouse hover:
.fade-in {
width: 100px;
height: 100px;
background-color: red;
opacity: 0.00;
cursor: pointer;
transition: 1s all ease-in-out;
-moz-transition: 1s all ease-in-out;
-webkit-transition: 1s all ease-in-out;
}
.fade-in:hover {
opacity: 1.00;
}
This CSS snippet utilizes the transition
and opacity
properties that we covered above, allowing for the element to fade in after a quarter of a second on mouse hover by defining a rule with the :hover
pseudo-class. When moving the mouse pointer away from the boundaries of the .fade-in
element, the element will fade out.
You could apply these same rules to any type of HTML element, including text elements, images, backgrounds, and so forth.
Fade In Text on Hover
A fade in effect on a text element could be added with the following CSS code:
.fade-in {
font-size: 100px;
color: white;
opacity: 0.00;
transition: 1s all ease-in-out;
-moz-transition: 1s all ease-in-out;
-webkit-transition: 1s all ease-in-out;
}
.fade-in:hover {
opacity: 1.00;
}
The above code takes an HTML element with class .fade-in
and gives it a zero opacity, meaning it's fully transparent. When hovering over the element with your mouse, the opacity value will change to 1, meaning the element is fully opaque. This transition will occur over a one second time span.
Make sure to add your HTML element to the page:
<div class="fade-in">Hello There!</div>
Here is the outcome of the fade in text with CSS:
Fade In Image on Hover
You could apply a fade in transition to an image displayed on a web page with the following CSS:
.fade-in {
opacity: 0.00;
transition: opacity 1s;
-moz-transition: opacity 1s;
-webkit-transition: opacity 1s;
}
.fade-in:hover {
opacity: 1.00;
}
This is shorted a bit from the previous example. Since we don't need to worry about font size or color, those rules are omitted. As you can see, you can add transitions to many different elements.
And the following HTML code:
<img class="fade-in" src="/path/to/image.jpg" />
The outcome is an image that fades in over a one second duration on mouse hover:
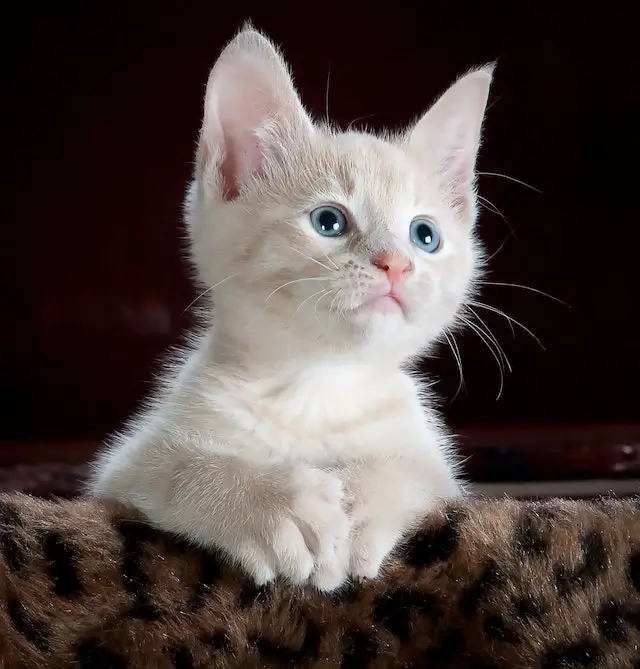
Fade In On Scroll
To create a fade in animation when scrolling an element into the viewport, we can create a similar set of CSS rules that we did above. The only exception here is we'll swap the :hover
pseudo-class with an .active
class:
.fade-in {
width: 100px;
height: 100px;
background-color: red;
opacity: 0.00;
transition: 1s all ease-in-out;
-moz-transition: 1s all ease-in-out;
-webkit-transition: 1s all ease-in-out;
}
.fade-in.active {
opacity: 1.00;
}
Then, we'll create a quick jQuery function that determines our current scroll position and adds the .active
class to the .fade-in
element once it has entered the viewport:
$(document).ready(function() {
$(window).on("scroll", function() {
var scroll_pos = $(window).scrollTop();
var element_pos = $(".fade-in").offset().top;
if (scroll_pos >= element_pos) {
$(".fade-in").addClass("active");
}
});
});
The scroll_pos
variable contains the value of the top scroll position in the viewport and the element_pos
variable contains the value of our HTML element. Once the scroll position is greater than or equal to the HTML element's top edge position, the element will fade in.
Conclusion
Now, you should be a master with CSS fade in transitions, right? Play around with the code and get creative with it. That's the true way to learn!
The great thing about most CSS transitions is that very little code is required to do simple yet impressive things that are both effective and visually stunning.
For more advanced animation features, check out the next post on creating a successful CSS keyframe animation.
Written by: J. Rowe, Web Developer
Last Updated: July 08, 2023Created: March 10, 2020