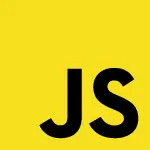
JavaScript
Unpeel the layers of JavaScript with these informative tutorials and code examples!
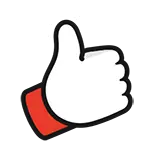
3 Ways to Check if a Value is a Number in JavaScript
In this tutorial, we'll explore three different ways to check if a value is a number in JavaScript.
JavaScript Array: Push, Pop, Shift, Unshift & Splice
Learn how to alter and manipulate JavaScript arrays when using the push(), pop(), shift(), unshift(), and splice() methods.
How to Create A 2D Tile-Based Game with JavaScript
Learn to build a 2D tile-based game with JavaScript using no third-party libraries. Great for beginner to intermediate developers!
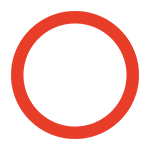
Check if a String Contains a Substring in JavaScript
Learn six effective methods to check if a string contains a substring in JavaScript, including examples and detailed explanations.
How to Clone Objects in JavaScript: A Complete Guide
Learn how to clone objects, arrays, and special data types in JavaScript with this complete shallow and deep cloning guide.
Checking File Size on Upload in JavaScript
Learn how to check file size on upload in JavaScript with this comprehensive client-side tutorial. Improve user experience easily!
Create A Single Page Application with JavaScript
Learn how to build a single-page application with JavaScript. Step-by-step guide for creating dynamic web experiences efficiently.
The 7 JavaScript Data Types with Examples
Learn about the seven JavaScript data types and how you can apply them in your web applications. Code examples included.
Get the Client's Timezone & Offset in JavaScript
Explore two effective methods for retrieving the client's timezone and offset using JavaScript and the International API.
How To Slugify A String with JavaScript
This tutorial will show you how to convert any text string into a URL-friendly slug using JavaScript.
Difference Between let and var in JavaScript
Discover the difference between using the let and var variable declaration keywords in JavaScript.
setTimeout() vs. setInterval() in JavaScript
Explore the differences between the setTimeout() and setInterval() time-based functions in JavaScript.
Determine if a Date is Today's Date Using JavaScript
Determine if a specified date is today's date with the JavaScript Date object.
Location Reload: How to Reload a Page in JavaScript
Learn how to reload a page programmatically with JavaScript and the Location.reload() method.
How to Wait for the DOM Ready Event in JavaScript
Learn how to wait for the DOM ready event in JavaScript.
How to Get URL Parameters in JavaScript
Get URL parameters with ease using the JavaScript URLSearchParams interface. Parse and manipulate URL parameters.
How to Create Multiline Strings in JavaScript
This tutorial will show you how to create clean multiline strings in JavaScript, making your code more readable.
How to Get the Current Timestamp in JavaScript
Learn what timestamps are and how to retrieve the current timestamp in JavaScript using the Date object.
Validate User Input with Regex in JavaScript
This tutorial will teach you how to validate user input with regular expressions in JavaScript.
Remove the Last Character From A JavaScript String
This tutorial will show you three different and simple methods for removing the last character of a string in JavaScript.
Format Numbers as Currency Strings in JavaScript
In this tutorial, you'll learn how to format numbers as currency strings in JavaScript using the Internationalization API.
How to Get the Last Array Element with JavaScript
In this quick tutorial, you'll learn a few different methods for retrieving the last element of an array in JavaScript.
How to Sort an Array of Objects in JavaScript
In this tutorial, we'll explore multiple ways to sort an array of objects in JavaScript and create a custom function.
How to Crop Image Whitespace with JavaScript
In this tutorial, you'll learn how to crop an image with JavaScript, removing unnecessary whitespace from the image's edges progra
Copy to the Clipboard in JavaScript & Clipboard API
In this tutorial, you'll learn how to copy to the clipboard in JavaScript with the Clipboard API.
JavaScript Date Difference Calculations
Learn how to calculate the difference between two dates using vanilla JavaScript.
How to Find & Remove Duplicates in JavaScript Arrays
In this tutorial, we'll discover how to find duplicates within an array in JavaScript, as well as how to remove the duplicates.
Filtering Array Values & Objects with JavaScript
This article covers the filter() method and how to filter values and objects using JavaScript.
How to Google Translate with JavaScript
Learn how to implement the Google Translate in JavaScript for translating page content into many different languages.
How to Create a Simple Calculator with JavaScript
Learn how to create a simple calculator with JavaScript, CSS, and HTML, with the ability to add, subtract, multiply, and divide.
jQuery Table Sorting: Sort Data on Header Click
Learn how to sort table data without plugins. All you need is jQuery and a few custom functions to get started!
jQuery, AJAX & PHP Asynchronous Multiple File Upload
Learn how to chunk upload multiple files asynchronously in a single form submission using jQuery, AJAX, and PHP.
JavaScript Comments: Improving Code Readability
Learn how to comment in JavaScript, how comments are used, and why they're important.
Enable Smooth Scrolling with JavaScript
Learn how to smooth scroll with JavaScript. Clean scrolling animations with no third-party libraries required.
JavaScript String Replacement: Simple Text & Regex
This article covers text and regular expression string replacement in JavaScript, one of the most common JS string operations.
JavaScript Promises: Resolve & Reject Code Examples
JavaScript Promises are a way of dealing with asynchronous operations and results, either with a resolve or reject callback.
Web Push Notifications with Push API in JavaScript
This tutorial dives deep into implementing web push notifications with JavaScript and the Push API for the modern browser.
Create a Random Number Generator with JavaScript
Learn how to create a random number generator with JavaScript, including how to generate a random number within a specified range.
JavaScript Array Length Property: Getting & Setting
Learn how to get and set an array length in JavaScript, how to manipulate array elements and data, and how to empty arrays.
JavaScript includes() Method for Array Searching
The JavaScript includes() method determines whether or not an array contains a specific string or not, returning a boolean value.
JavaScript indexOf() Method for Arrays & Strings
Learn how to use the JavaScript indexof() method for returning array and string index values, character or word positions, etc.
Detecting Idle Browser Tabs: Page Visibility API
The Page Visibility API is a great resource for determining which of your browser windows or tabs are currently active or idle.
JavaScript Array map() Method: Arrays in JavaScript
Learn how to use the JavaScript Array map() method and how you can read, query, and transform array or page elements in an array.
How JavaScript Works: Engine, Runtime & Call Stack
Dive into a detailed overview of how JavaScript works, including details on the engine, runtime, call stack, and event queue.
JavaScript Cookies: Get, Set, Delete & Security
Learn how to get, set, and delete cookies with JavaScript, as well as important cookie security features to take advantage of.
The JavaScript forEach() Method for Array Looping
Learn how to use JavaScript's forEach() method to loop through array data and the benefits it provides versus an iterated loop.
How to Create a Simple Chat Room with JavaScript
This tutorial walks through the steps of creating a simple JavaScript chat room with websockets and two server-side technologies.
localStorage vs. sessionStorage & Web Storage API
localStorage and sessionStorage are part of the Web Storage API, allowing you to save strings and key/value pairs locally.
How to Parse & Stringify JSON Objects in JavaScript
Learn efficient data handling in JavaScript with JSON.parse() and JSON.stringify(). Master techniques for dynamic web development.
JavaScript Equalizer Display with Web Audio API
Create a JavaScript Equalizer that utilizes the Web Audio API, a high-level JavaScript API for processing and synthesizing audio.